Order Management
Zuletzt bearbeitet am: 08.03.2024
Introduction
Order Management is part of both plugins: Klarna Payments and Klarna Checkout. The Order Management module bundles components needed by both plugins: The handling of Klarna orders within the Shopware backend.
Usage and operation
Klarna tab in the order
In the Klarna tab in the order details you will find an overview of the Klarna order as well as various actions.
The Klarna tab is only displayed for orders paid with a Klarna payment method.
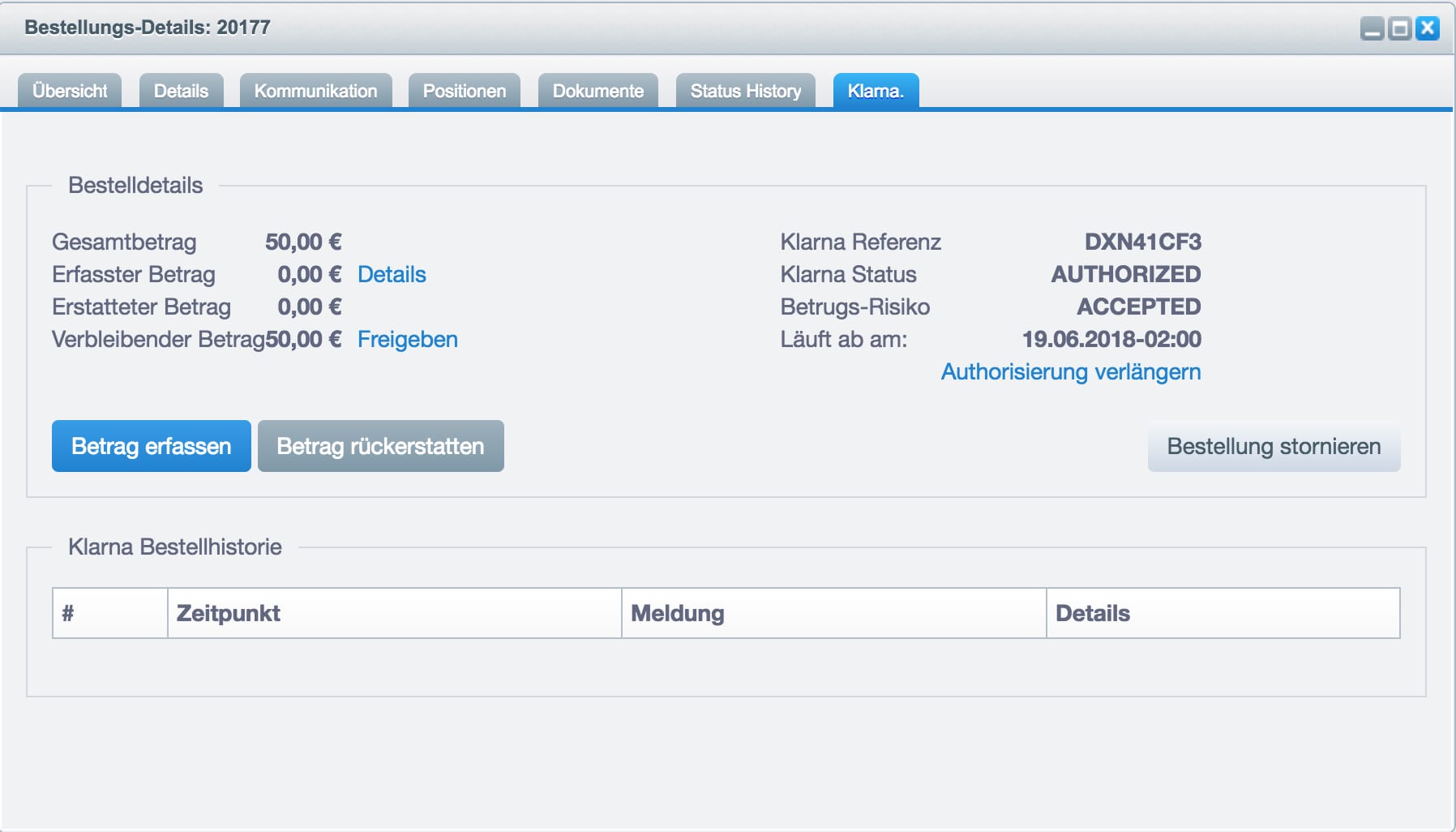
name | description |
---|---|
Total amount | The total amount of the order. |
Amount captured | The amount that has already been captured (debited). |
Amount refunded | The amount that has been refunded. |
Remaining amount | The amount that can still be captured (amount not yet debited). |
Klarna Reference | A Klarna internal ID to identify the order. |
Klarna Status | The order status that is stored at Klarna. |
Fraud Risk | The Klarna Risk Check status. |
Expires on | The date on which the authorization expires. From that date on, the remaining amount can no longer be captured. |
Capture amount manually
You can capture an amount by clicking on the "Capture amount" button. A new window will be displayed where you can specify the amount, the positions and a comment.
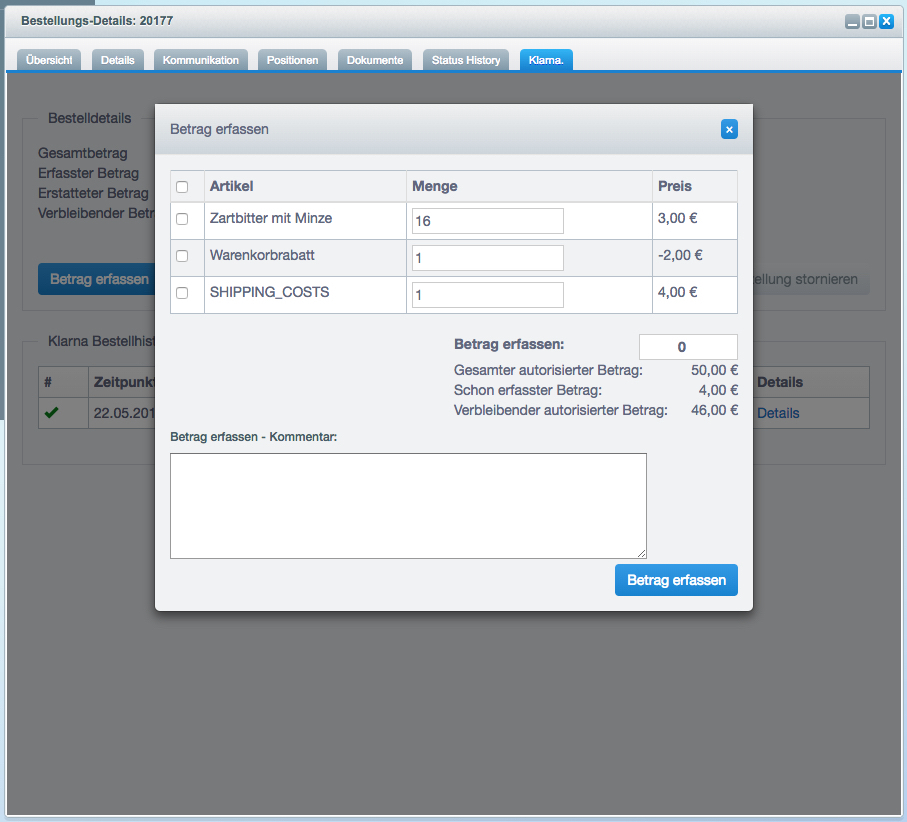
The selection of items is optional, you can also enter an amount manually. In this case, however, Klarna does not receive the information about which items have already been captured. Therefore, we always recommend to make the entry via the item selection.
The comment is also optional and serves to clarify why an amount was captured. For example, "Item XY was not captured because it was out of stock."
Once you have selected the desired items and the desired amount, you can capture the amount by clicking "Capture Amount".
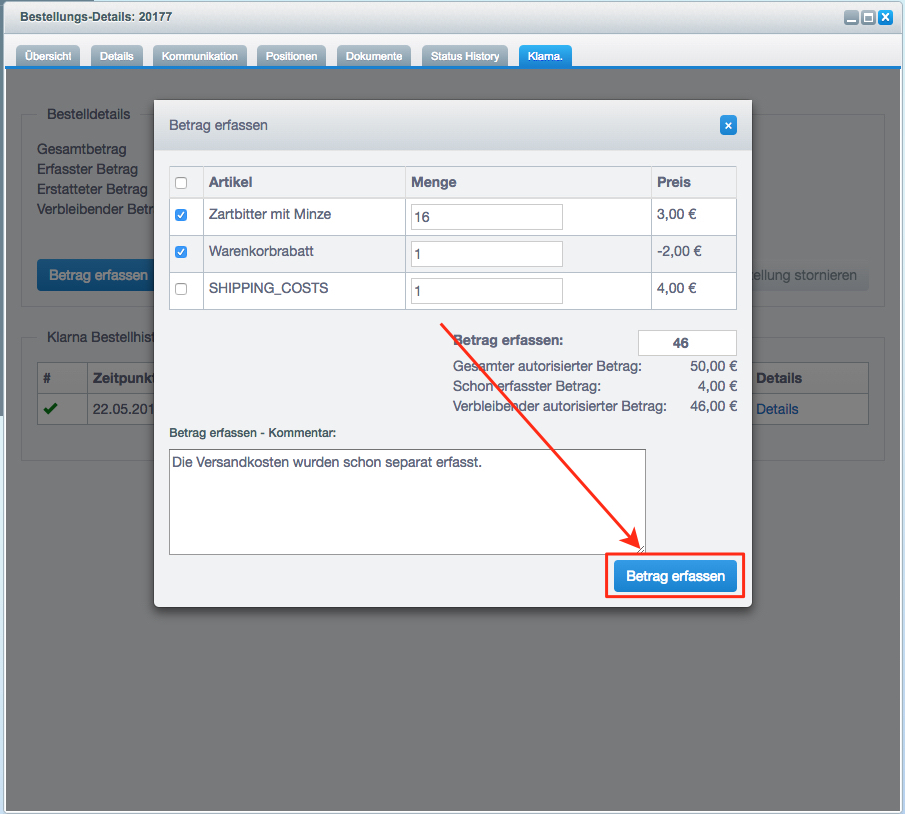
You will now be asked if you want to perform the capture.
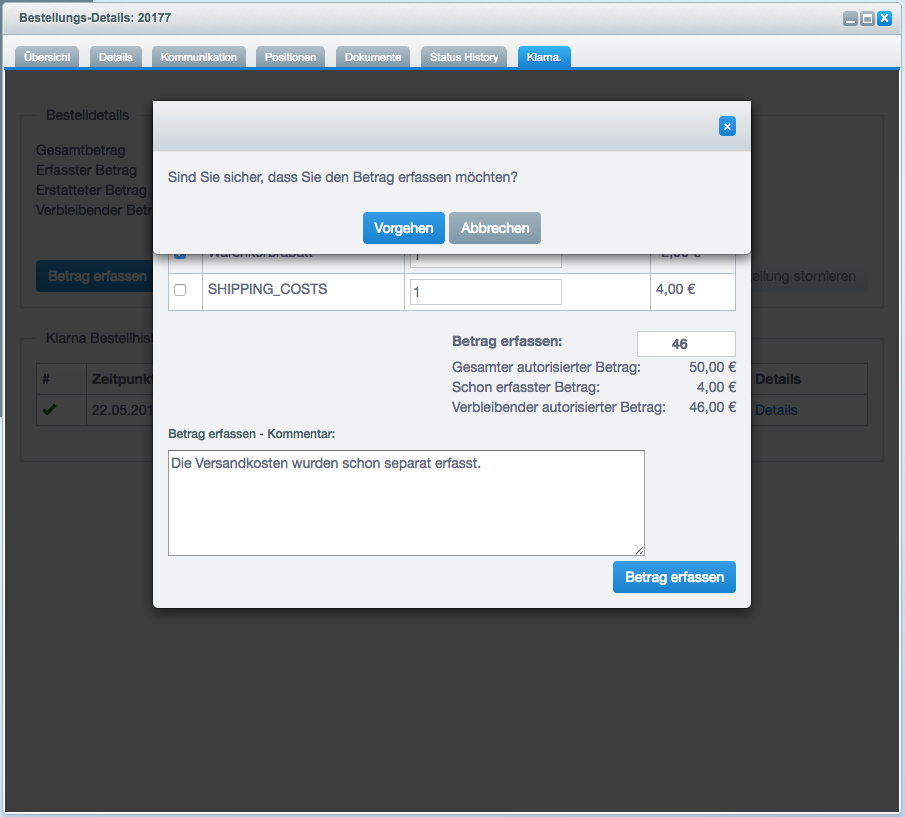
Confirm the action by clicking on the "Proceed" button. In case of error or success, a corresponding message will be displayed.
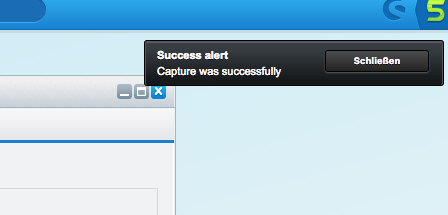
Refund amount manually
You can refund an amount by clicking on the "Refund amount" button. You will now see a new window where you can specify the amount, items and a comment.
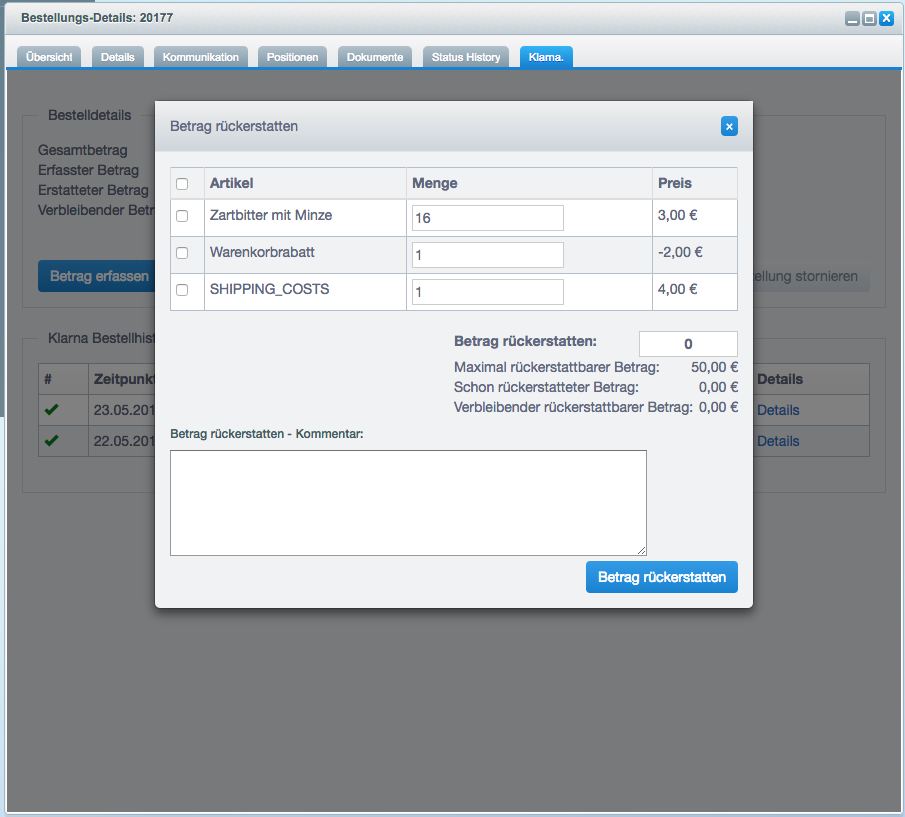
The selection of items is optional, you can also enter an amount manually. However, in this case Klarna will not receive the information which items have been refunded. Therefore, we always recommend to make the refund via the item selection.
The comment is also optional and serves to clarify why an amount was refunded. For example, "Item XY was refunded because it was out of stock."
Once you have selected the items you want and the amount you want, you can refund the amount by clicking "Refund Amount".
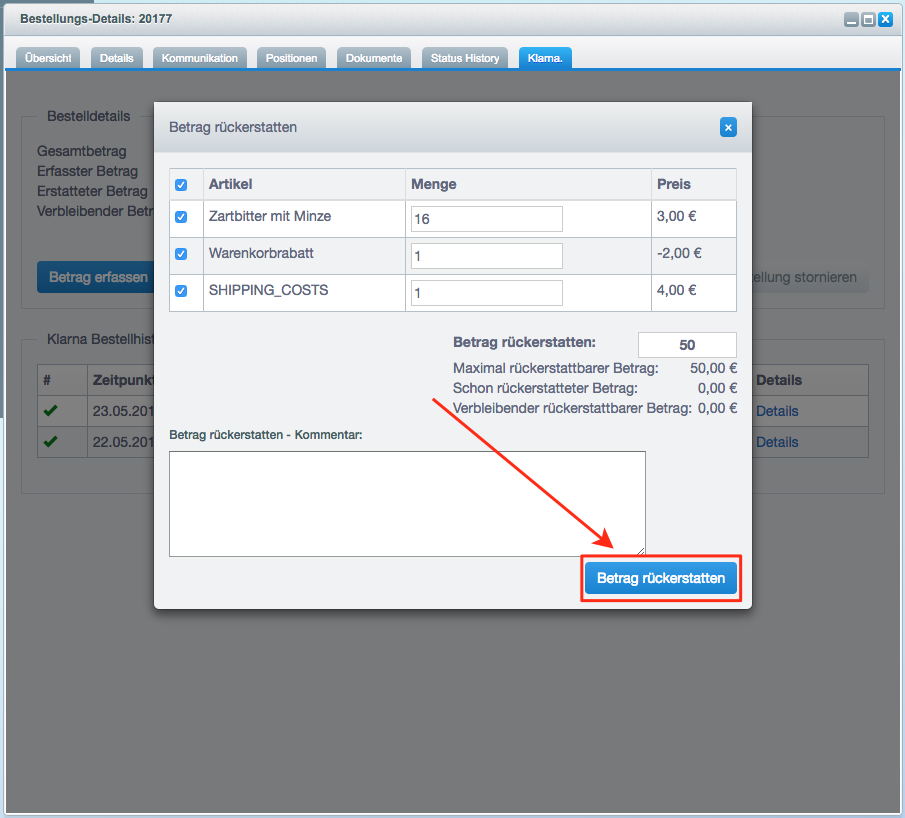
You will now be asked if you would like to refund.
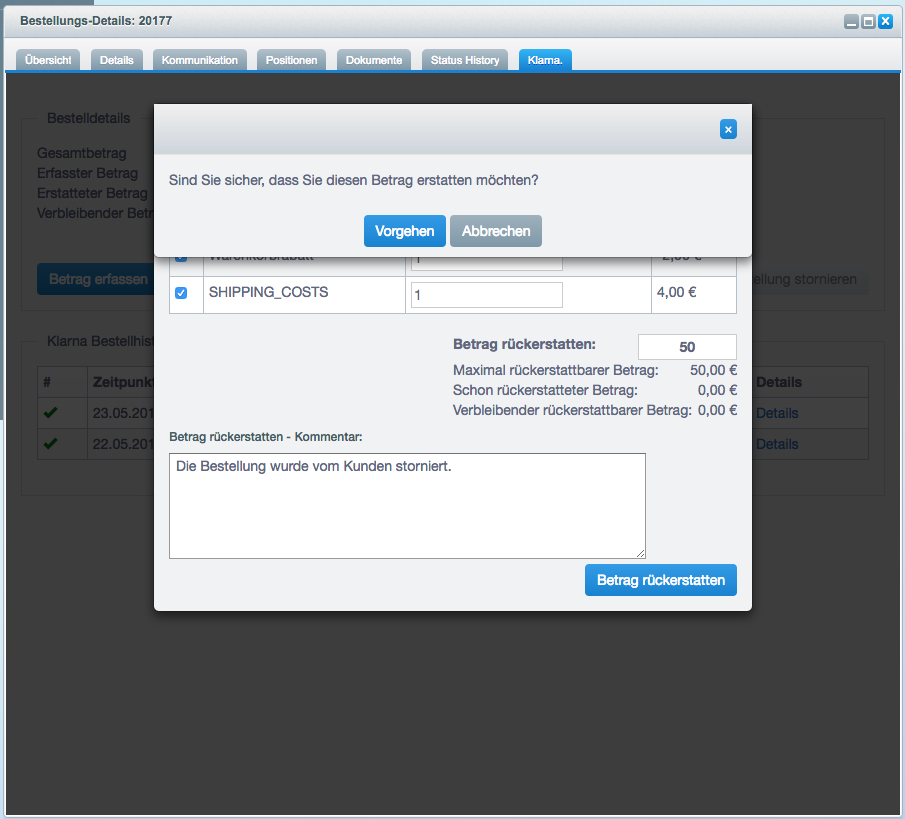
Confirm the action by clicking on the "Proceed" button. In case of error or success, a corresponding message will be displayed.
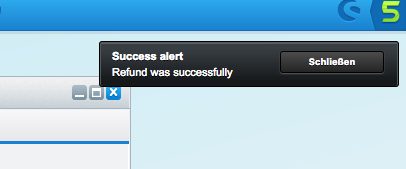
Manually extend authorization
You can extend the authorization of a payment by clicking on the "Extend Authorization" link.
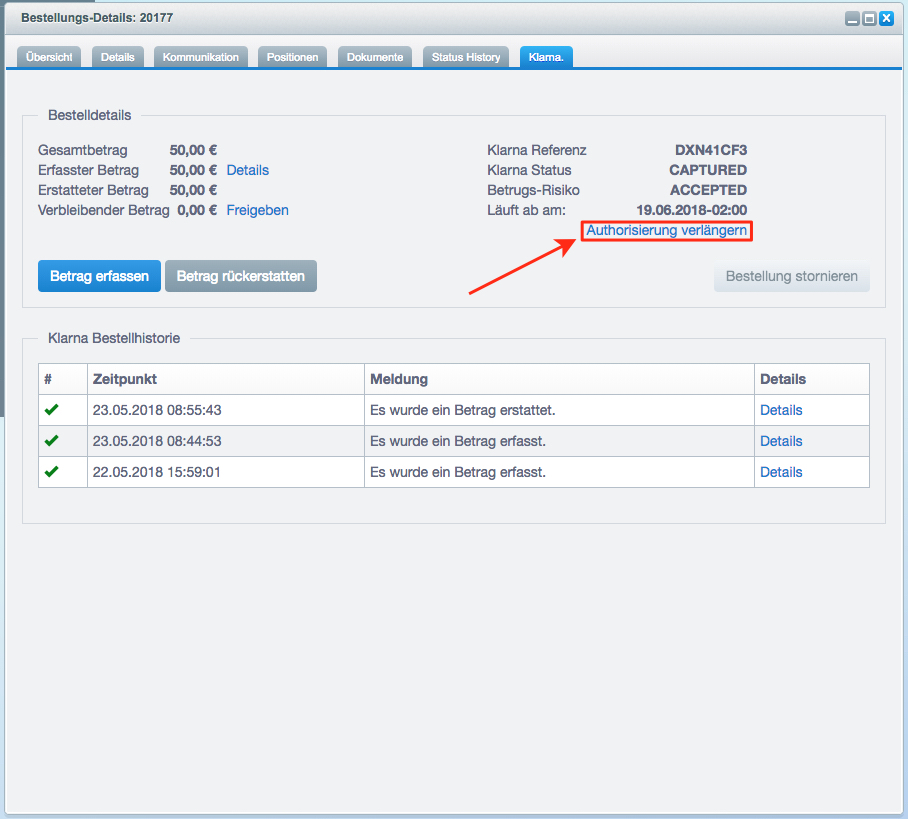
You will now be asked if you really want to extend the authorization.
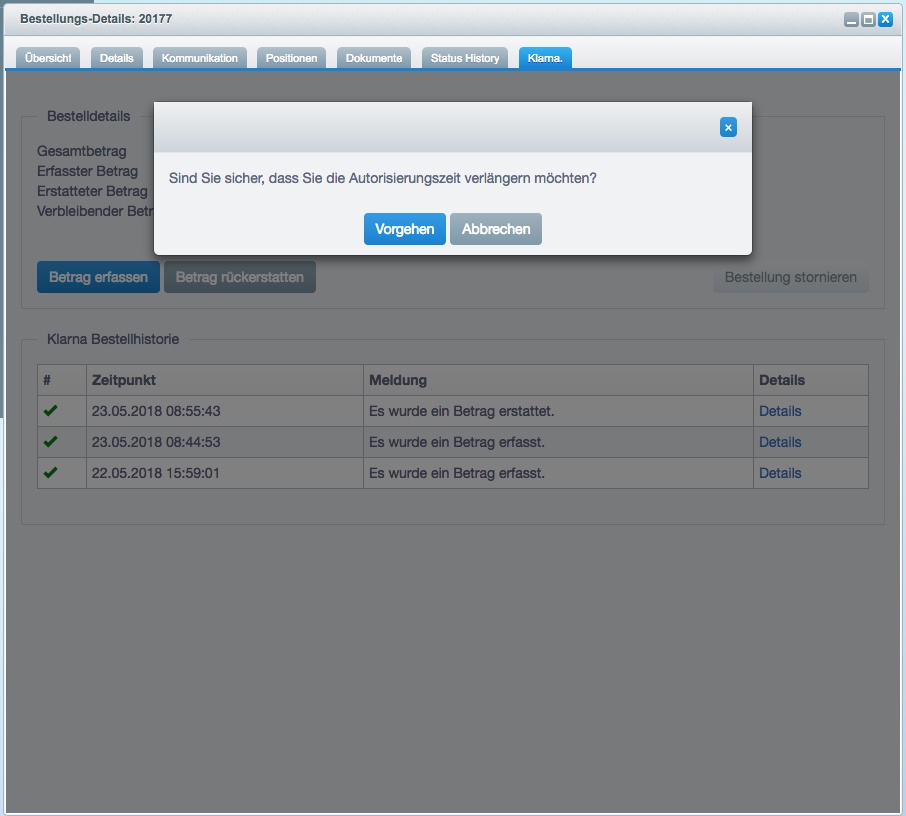
Confirm the action by clicking on the "Proceed" button. In case of error or success, a corresponding message will be displayed.
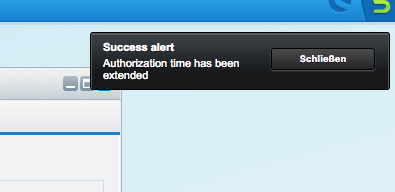
Release remaining amount manually
You can release (unauthorize) the remaining amount by clicking on the "Release" link.
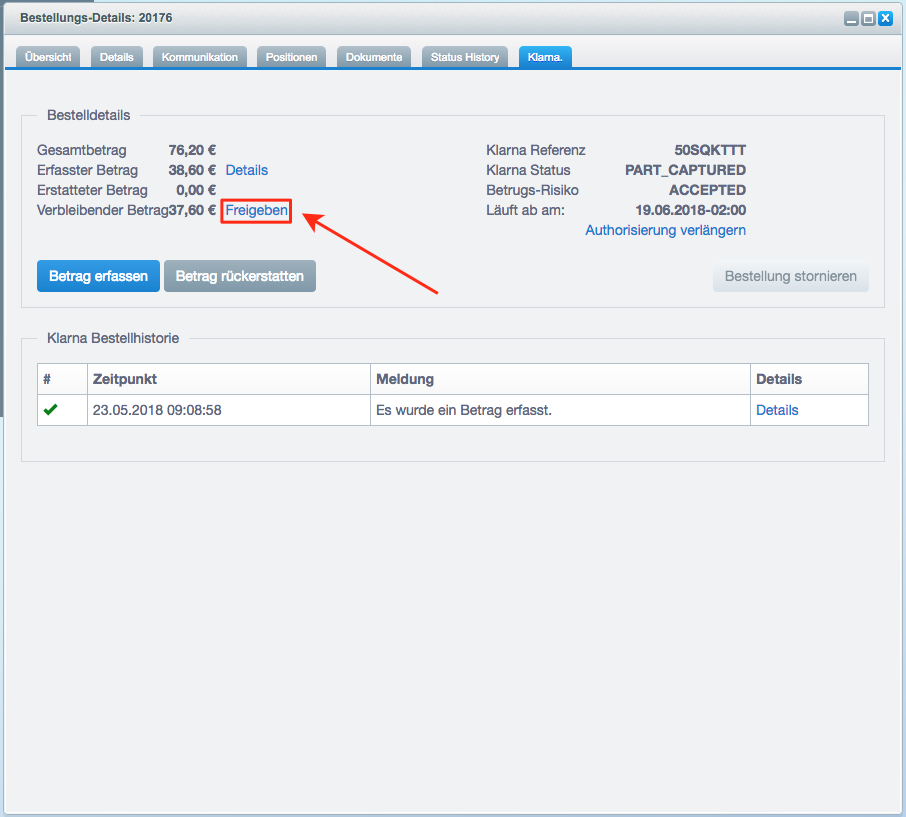
You will now be asked if you really want to release the remaining amount.
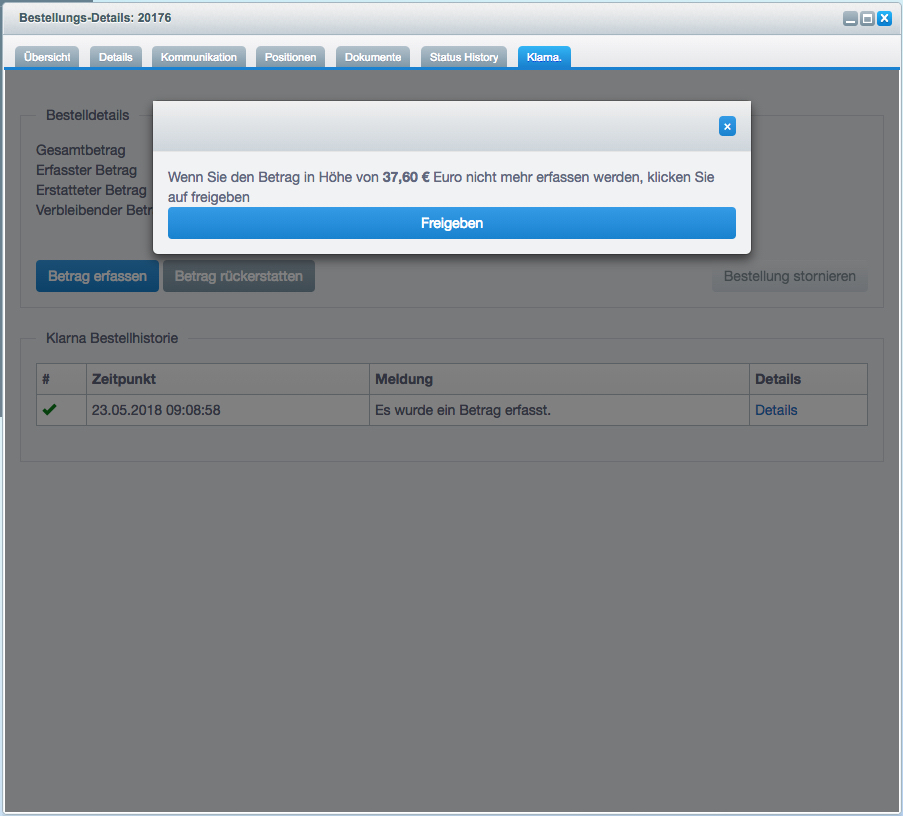
Confirm the action by clicking on the "Release" button. In case of error or success, a corresponding message will be displayed.
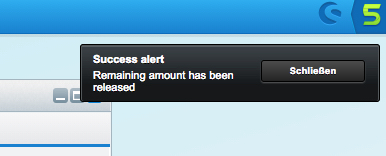
Resend invoice manually
You can resend an invoice per captured amount.
To get an overview of all recorded amounts, click on the Details
link.
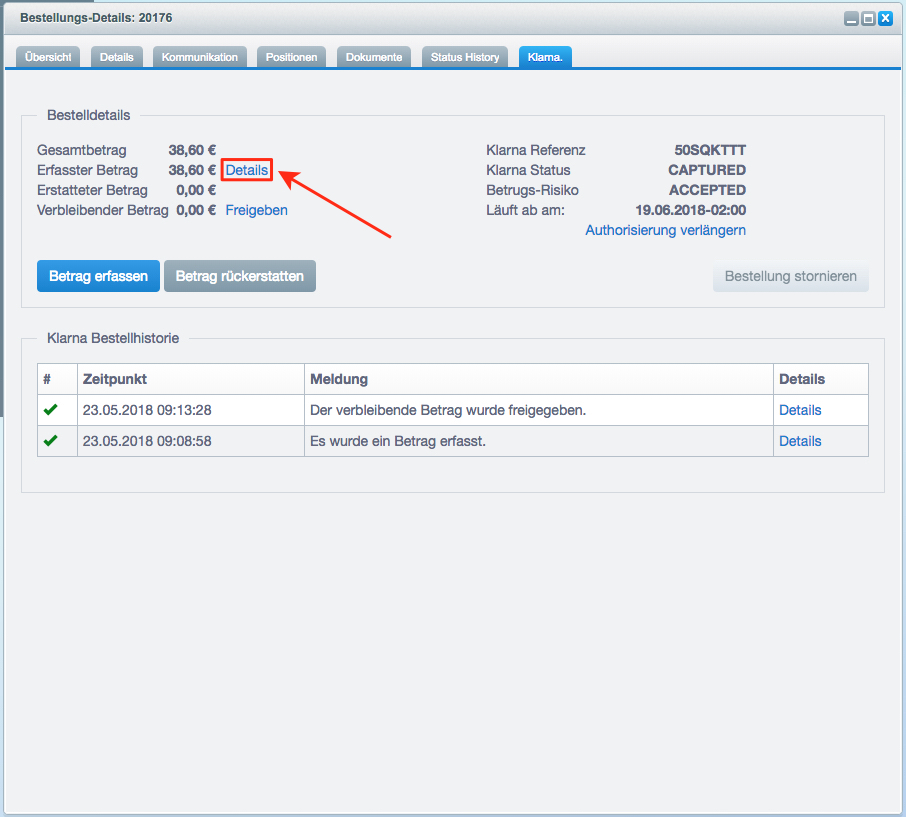
You will now see an overview of all the amounts captured and a "Resend invoice" button for each. If you want to resend the invoice for a captured amount, click the "Resend invoice" button under the captured amount.
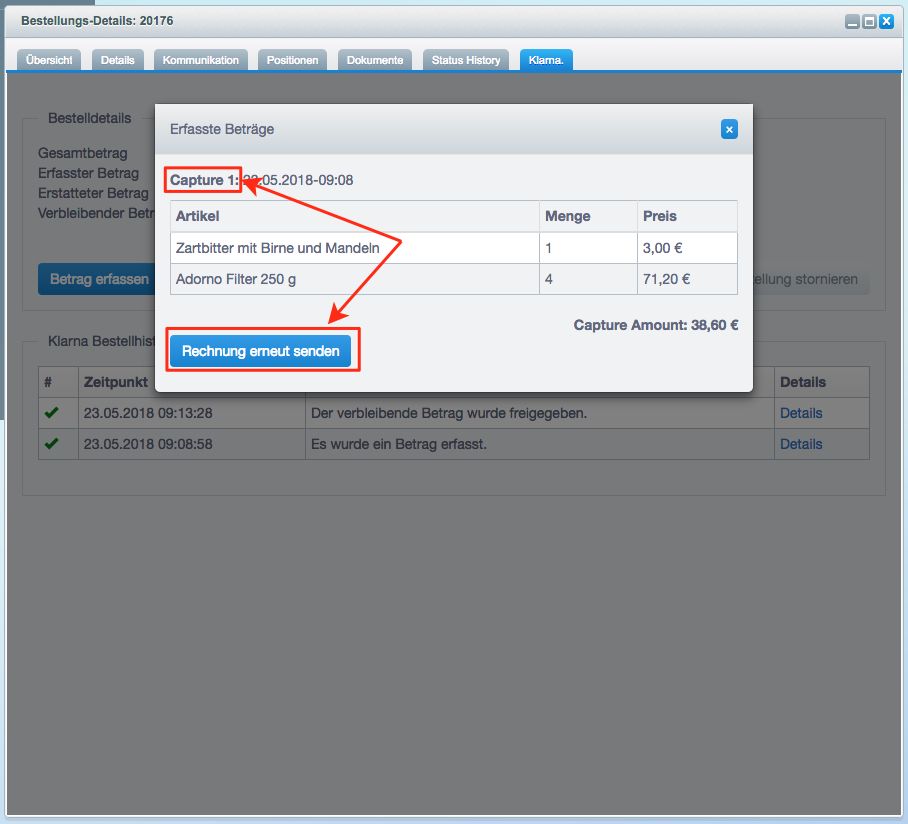
You will now be asked if you really want to resend the invoice.
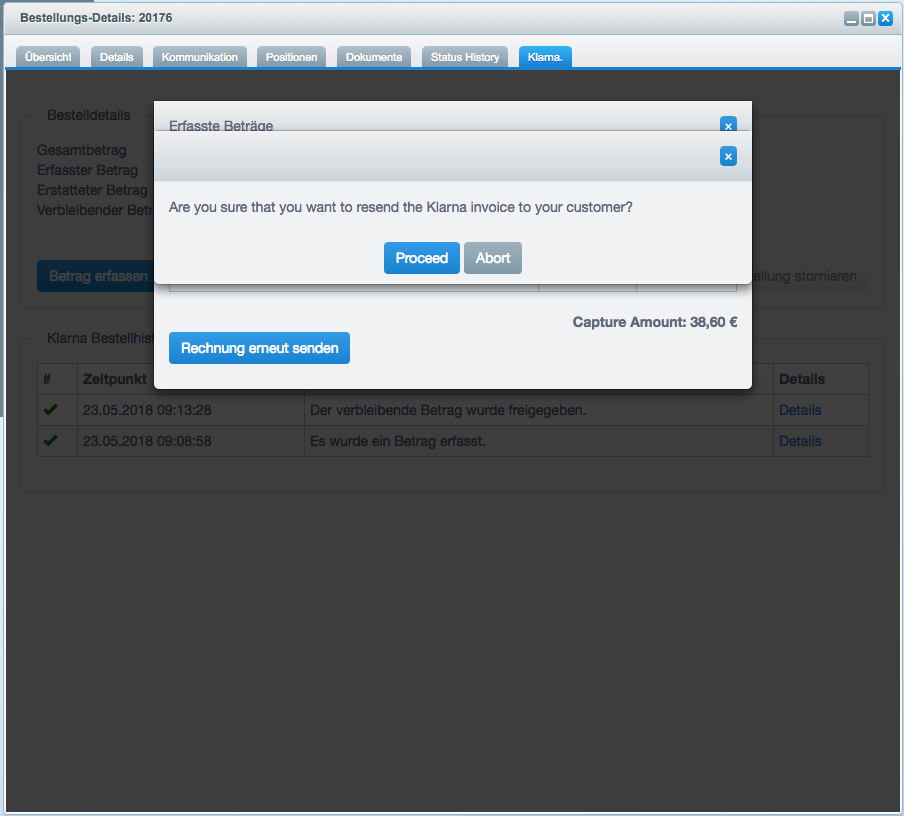
Confirm the action by clicking on the "Proceed" button. In case of error or success, a corresponding message will be displayed.
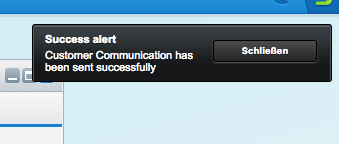
Order history
The order history lists all actions that were performed for the order.
Column | Description |
---|---|
# | The column tells whether the action was successful or not. A green tick indicates that the action was successful. A red X says that the action failed. |
Time | The time when the action was performed. |
Message | The action that was performed. |
Details | A link which opens a dialog with more information. |
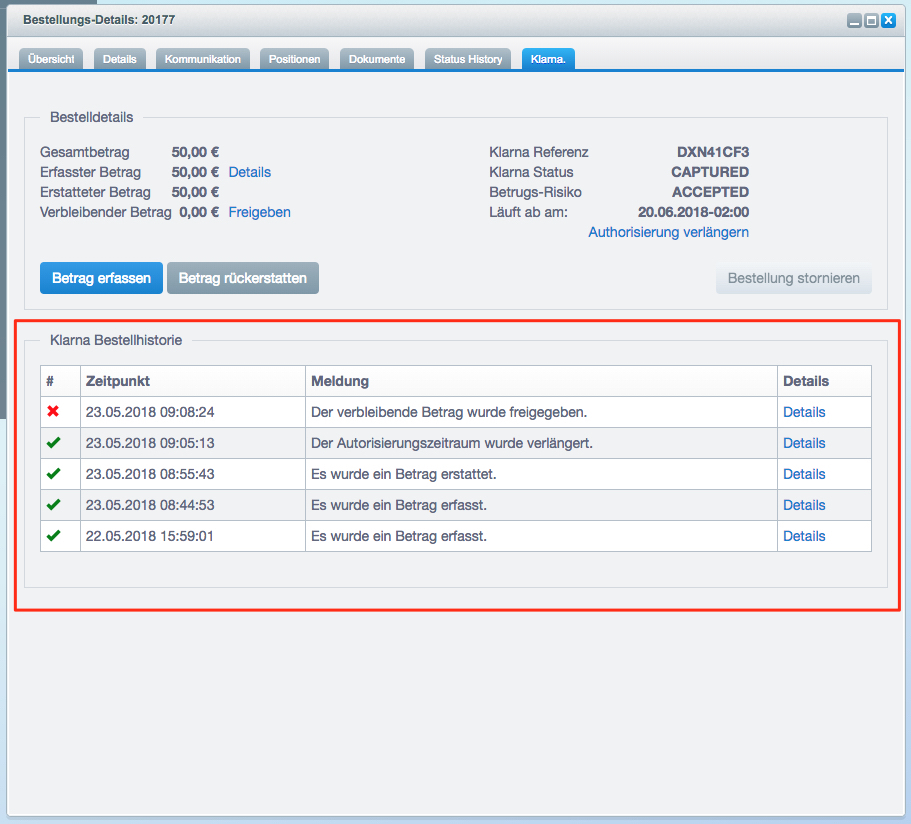
The following messages may appear:
Message | Description/Information |
---|---|
An amount was captured. | Which amount was captured can be seen in the "Details" dialog. |
An amount has been refunded. | The amount refunded can be seen in the "Details" dialog. |
Order details have been updated. | The order has been updated. For example, an item was removed. |
The authorization period has been extended. | Will be executed when clicking the "Extend Authorization" link within the Klarna tab. |
The remaining amount has been released. | The remaining (not yet entered amount) has been released and therefore cannot be entered anymore. |
Order has been canceled. | The order has been cancelled at Klarna and can no longer be entered. |
Example of a dialog with further information about an action.
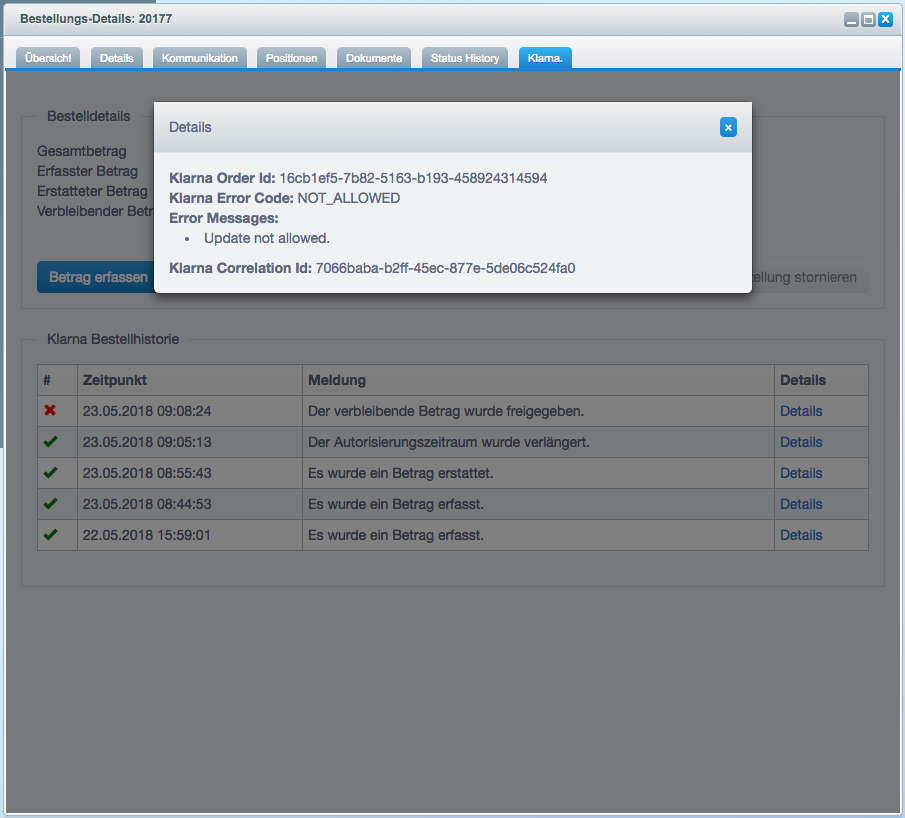
Cancel order manually
An order can be canceled only if no amount has been entered yet.
The cancellation of an order cannot be undone.
To cancel an order click the "cancel order" button.
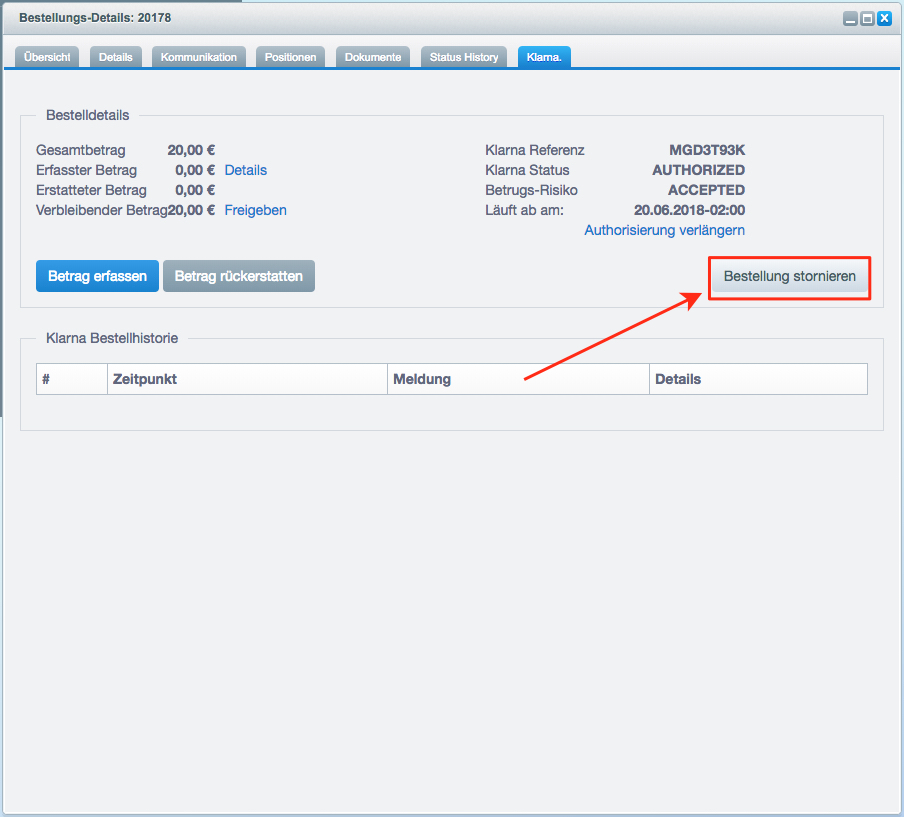
You will now be asked if you really want to cancel the order.
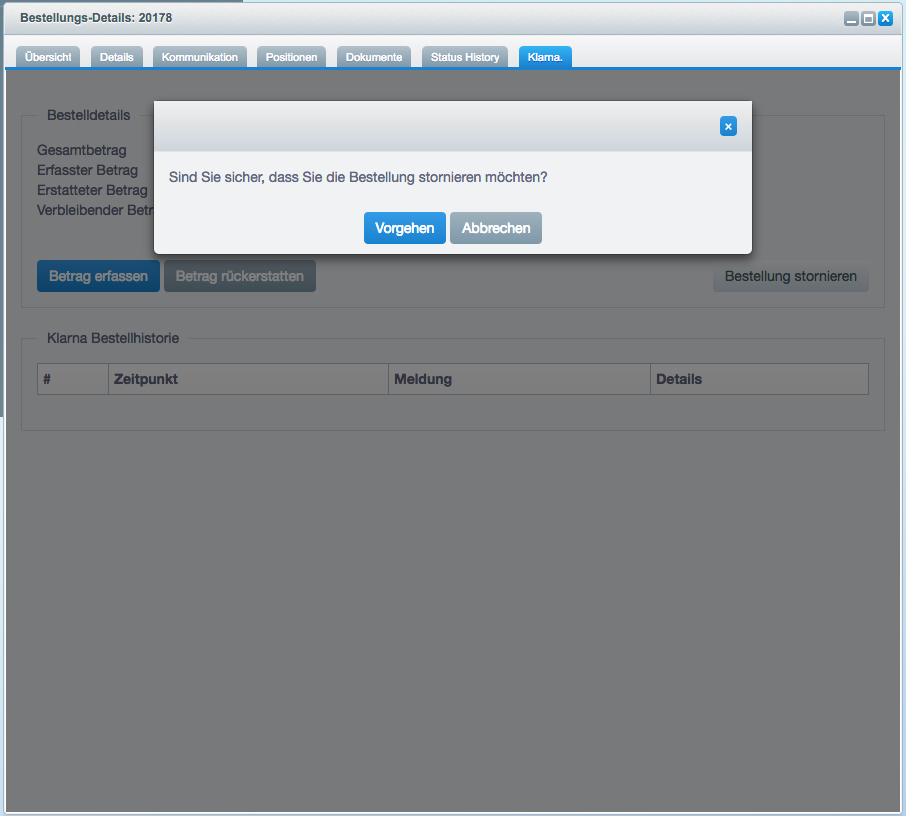
Confirm the action by clicking on the "Proceed" button. In case of error or success, a corresponding message will be displayed.
Once an order is cancelled, no further action can be performed on that order.
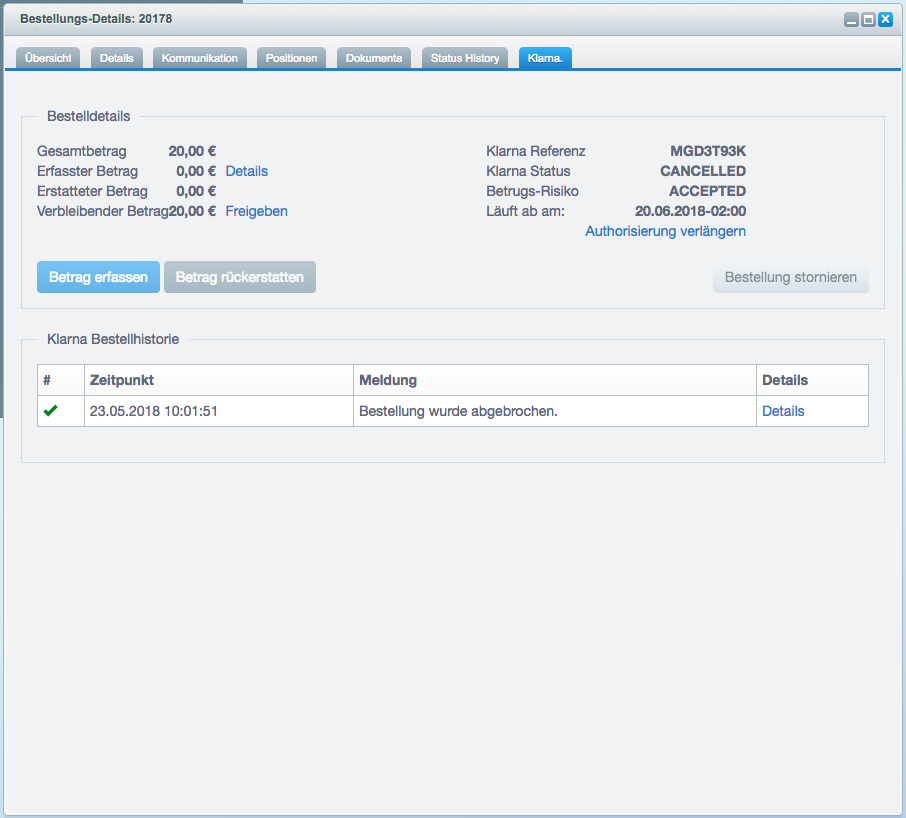
Automatic changes
Defined changes to an order will automatically trigger actions at Klarna.
Payment status
The payment status is automatically set by Klarna for certain actions.
Action | Payment status |
---|---|
Partial capture | Partially paid |
Partial refund | Re-crediting |
Full capture | Completely paid |
Full refund | Re-crediting |
Address changes
When changing the address of an order in the backend, the new data is first transferred to Klarna. Only if Klarna accepts the new address, it will be updated in Shopware. This ensures that the new address has been accepted by the Klarna risk check.
Please note that a change will not be accepted by Klarna once an amount of the order has been captured.
Changes to the order
When changes are made to an order (e.g. positions), the new data is first transferred to Klarna. Only if Klarna accepts the new order, it will be updated in Shopware. This ensures that the new data has been accepted by the Klarna risk check.
Changes that increase the Authorized Amount are generally rejected.
Please note that a change is not accepted by Klarna once an amount of the order has been captured.
Shipment number
When changing the shipment number, it will be transferred to Klarna. For multiple shipment numbers, please refer to the respective plugin configuration for shipment number separator.
The shipment number is automatically transmitted to Klarna during captures.
Change payment type
Changing the payment type from one Klarna payment type to another can only be done if no amount has been entered yet. In this case the order will be automatically cancelled by Klarna.
The payment type cannot be changed from one Klarna payment type to another Klarna payment type.
Delete order
An order can be deleted only if no amount has been captured yet. In this case the order will be automatically cancelled by Klarna.
Extending the plugin
Order Management components are designed to be used not only by both Klarna plugins but also by your individual plugin. For easier control we have provided facades.
Partly the components need the Klarna-Order-Id. This is stored as the transaction ID of an order in Shopware.
Facades
Retrieve order
To retrieve an order, you need the BestitKlarnaOrderManagement\Components\Facade\Order
facade.
Through it, you can call the get
method, which retrieves an order from Klarna.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Order as OrderFacade;
class Example
{
/** @var OrderFacade */
protected $orderFacade;
public function __construct(OrderFacade $orderFacade)
{
$this->orderFacade = $orderFacade;
}
public function example()
{
$klarnaResponse = $this->orderFacade->get('klarna-order-id');
}
}
Capture amount
To capture an amount, you need the BestitKlarnaOrderManagement\Components\Facade\Capture
facade.
Through it, you can call the create
method, which captures an amount.
You can also give the method the items as JSON, a comment and shipping information.
The positions JSON must be formatted so that it can be deserialized into an array of BestitKlarnaOrderManagement\Components\Api\Model\LineItem
.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Capture as CaptureFacade;
class Example
{
/** @var CaptureFacade */
protected $captureFacade;
public function __construct(CaptureFacade $captureFacade)
{
$this->captureFacade = $captureFacade;
}
public function example()
{
$betragInCents = 500;
$klarnaResponse = $this->captureFacade->create('klarna-order-id', $betragInCents);
}
}
Refund amount
To refund an amount, you need the BestitKlarnaOrderManagement\Components\Facade\Refund
facade.
Through it, you can call the create
method, which will refund an amount.
You can give the method the items as JSON)and a comment.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Refund as RefundFacade;
class Example
{
/** @var RefundFacade */
protected $refundFacade;
public function __construct(RefundFacade $refundFacade)
{
$this->refundFacade = $refundFacade;
}
public function example()
{
$betragInCents = 500;
$klarnaResponse = $this->refundFacade->create('klarna-order-id', $betragInCents);
}
}
Update order
To update an order, you need the BestitKlarnaOrderManagement\Components\Facade\Order
facade.
Through it, you can call the updateOrder
method, which updates an order.
This method needs the Klarna order id, the new total amount of the order and an Array of BestitKlarnaOrderManagement\Components\Api\Model\LineItem
with all items.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Api\Model\LineItem;
use BestitKlarnaOrderManagement\Components\Api\Model\ProductIdentifiers;
use BestitKlarnaOrderManagement\Components\Constants;
use BestitKlarnaOrderManagement\Components\Facade\Order as OrderFacade;
class Example
{
/** @var OrderFacade */
protected $orderFacade;
public function __construct(OrderFacade $orderFacade)
{
$this->orderFacade = $orderFacade;
}
public function example()
{
$lineItem = new LineItem();
$lineItem->type = Constants::KLARNA_LINE_ITEM_TYPE_PHYSICAL;
$lineItem->totalTaxAmount = 0;
$lineItem->totalAmount = 0;
$lineItem->quantity = 1;
$lineItem->name = 'Free article';
$lineItem->productIdentifiers = new ProductIdentifiers(); // ProductIdentifiers are blank intentionally
$lineItem->taxRate = 0;
$lineItem->totalDiscountAmount = 0;
$lineItem->unitPrice = 0;
$lineItem->quantityUnit = 'Pieces';
$lineItem->reference = 'SW1000';
$klarnaResponse = $this->orderFacade->updateOrder('klarna-order-id', 0, [$lineItem]);
}
}
Extend authorization
To extend an authorization, you need the BestitKlarnaOrderManagement\Components\Facade\Order
facade.
Through it, you can call the extendAuthTime
method which extends an authorization.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Order as OrderFacade;
class Example
{
/** @var OrderFacade */
protected $orderFacade;
public function __construct(OrderFacade $orderFacade)
{
$this->orderFacade = $orderFacade;
}
public function example()
{
$klarnaResponse = $this->orderFacade->extendAuthTime('klarna-order-id');
}
}
Release remaining amount
To release the remaining amount, you need the BestitKlarnaOrderManagement\Components\Facade\Order
facade.
Through it, you can call the releaseRemainingAmount
method which will release the remaining amount.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Order as OrderFacade;
class Example
{
/** @var OrderFacade */
protected $orderFacade;
public function __construct(OrderFacade $orderFacade)
{
$this->orderFacade = $orderFacade;
}
public function example()
{
$klarnaResponse = $this->orderFacade->releaseRemainingAmount('klarna-order-id');
}
}
Resend invoice
To resend an invoice, you need the BestitKlarnaOrdferManagement\Components\Facade\Capture
facade.
Through it, you can call the resend
method, which resends the invoice.
Since an invoice is created per captured amount, it is also possible to resend an invoice only for a specific captured amount.
This requires the capture id
which is supplied with the order-call.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Capture as CaptureFacade;
class Example
{
/** @var CaptureFacade */
protected $captureFacade;
public function __construct(CaptureFacade $captureFacade)
{
$this->captureFacade = $captureFacade;
}
public function example()
{
$klarnaResponse = $this->captureFacade->resend('klarna-order-id', 'capture-id');
}
}
Cancel order
To cancel an order, you need the BestitKlarnaOrderManagement\Components\Facade\Order
facade.
Through it, you can call the cancel
method which cancels the order.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Facade\Order as OrderFacade;
class Example
{
/** @var OrderFacade */
protected $orderFacade;
public function __construct(OrderFacade $orderFacade)
{
$this->orderFacade = $orderFacade;
}
public function example()
{
$klarnaResponse = $this->orderFacade->cancel('klarna-order-id');
}
}
Cents calculation
By default, Shopware uses decimal numbers as prices (e.g. "3.14"), while Klarna works with cents and thus with whole numbers (e.g. "314"). Therefore, the Shopware prices must be converted to cents before they can be transferred to Klarna.
Please note that the configuration Net orders consistently round to 2 digits
(under Configuration -> Basic settings -> Frontend -> Shopping cart / item details
) can lead to rounding errors. We recommend saving this option to the default Yes
.
PHP Calculation
With the standard PHP calculation no extensions are necessary. However, rounding errors may occur.
BcMath calculation
Alternatively, the PHP extension bcmath can be installed on the server. Fewer rounding errors occur here due to internal processing.
Custom calculation
To add a custom calculation, the Decorator Pattern can be used to overwrite or extend the PHP calculation.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Calculator\CalculatorInterface;
class Example implements CalculatorInterface
{
/** @var CalculatorInterface */
protected $decoratedCalculator;
public function __construct(CalculatorInterface $decoratedCalculator)
{
$this->decoratedCalculator = $decoratedCalculator;
}
public function isSupported()
{
return $this->decoratedCalculator->isSupported();
}
public function toCents($amount)
{
return $amount * 100;
}
public function toMajorUnit($amount)
{
return $this->decoratedCalculator->toMajorUnit($amount);
}
}
In the service.xml
the new service must then be marked as a decorator:
<service
class="App\Example\Example"
id="app.example.example"
decorates="bestit_klarna_order_management.components.calculator.php_calculator">
<argument type="service" id="app.example.example.inner"/>
</service>
Position mode
Shopware stores a mode for each position, a kind of internal id, for the differentiation of the position type (e.g. article, discount or voucher). Klarna does not know these internal ids, and so they have to be converted to understandable types for Klarna.
For this we have created a Converter
class for each mode, which convert the Shopware mode to a Klarna type.
All Converter
must implement the BestitKlarnaOrderManagement\Components\Converter\ModeInterface
.
Modify converter
In the example, the Converter
of mode "2" (Shopware discount voucher) is modified so that it always returns the type gift_card
.
<?php
namespace App\Example;
use BestitKlarnaOrderManagement\Components\Constants;
use BestitKlarnaOrderManagement\Components\Converter\ModeInterface;
use BestitKlarnaOrderManagement\Components\Exception\NoSupportedModeException;
class Example implements ModeInterface
{
/** @var ModeInterface */
protected $decoratedConverter;
public function __construct(ModeInterface $decoratedConverter)
{
$this->decoratedConverter = $decoratedConverter;
}
public function isSupported($mode)
{
return $this->decoratedConverter->isSupported($mode);
}
public function convert($mode, $price = null)
{
if (!$this->isSupported($mode)) {
throw new NoSupportedModeException();
}
return Constants::KLARNA_LINE_ITEM_TYPE_GIFT_CARD;
}
}
In the service.xml
the new service must then be marked as a decorator:
<service
class="App\Example\Example"
id="app.example.example"
decorates="bestit_klarna_order_management.components.converter.voucher">
<argument type="service" id="app.example.example.inner"/>
</service>
Other
Automatic Klarna actions
By changing the order status, automatic actions can be performed by the plugin. See also the plugin configuration for this.
The automatic actions can only be performed by the plugin if the order update is saved via Doctrine ORM. Direct changes in the database cannot be taken into account for technical reasons.
Automatic capture
When the order status changes to the configured order status, a full capture is performed automatically.
Automatic refund
When the order status changes to the configured order status, a full refund will be executed automatically.